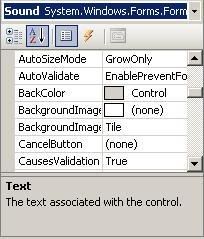
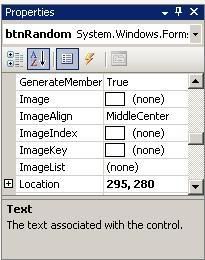
For the BackgroundImage, we can also set the image to be Tiled or Stretched however we like.
We also learned that we could change the images as the program runs. For example to set the button image to something else when it is clicked, we can write inside the Button_Click() subroutine:
Button.Image = Image.FromFile("nameofnewimagefile.jpg")
If we place our image files in the debug folder we can simply write the name of the file without having to put the entire path:
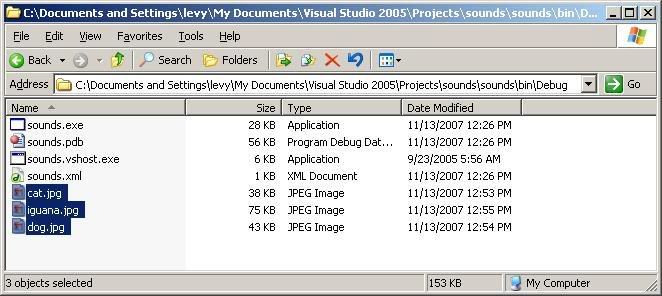
Suppose we want to write a program that will display pictures of different albums depending on which button is clicked. An example is below:

We could use the following code:
Private Sub Btncat_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles btnCat.Click
Me.BackgroundImage = Image.FromFile("cat.jpg")
Me.BackgroundImageLayout = ImageLayout.Stretch
End Sub
Private Sub BtnDog_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles btnDog.Click
Me.BackgroundImage = Image.FromFile("dog.jpg")
Me.BackgroundImageLayout = ImageLayout.Stretch
End Sub
Private Sub BtnIguana_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles btnIguana.Click
Me.BackgroundImage = Image.FromFile("iguana.jpg")
Me.BackgroundImageLayout = ImageLayout.Stretch
End Sub
Private Sub BtnRandom_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles btnRandom.Click
Dim randnum As New Random, temp As Integer
temp = randnum.Next(1, 4)
Select Case temp
Case 1
Me.BackgroundImage = Image.FromFile("iguana.jpg")
Me.BackgroundImageLayout = ImageLayout.Stretch
Case 2
Me.BackgroundImage = Image.FromFile("cat.jpg")
Me.BackgroundImageLayout = ImageLayout.Stretch
Case 3
Me.BackgroundImage = Image.FromFile("dog.jpg")
Me.BackgroundImageLayout = ImageLayout.Stretch
End Select
End Sub
No comments:
Post a Comment